This post was updated on September 11th, 2020.
Update: Dell does now provide native WMI classes to manage BIOS passwords on newer models. For more information, see this post: Dell BIOS Password Management – WMI. For information on using the Dell PowerShell module to configure passwords on older models, continue reading this post.
This is the third post in my series on how to manage BIOS / Firmware passwords with PowerShell. Previously, I’ve discussed Lenovo BIOS Password Management and HP BIOS Password Management.
This script is similar to the previous scripts in that the goal was to have a script that could automatically set, change, or clear BIOS passwords while providing logging and optional user prompts. However, there are a few differences. In this post, I’ll cover the basics of how the script works.
The script can be downloaded from my GitHub: https://github.com/ConfigJon/Firmware-Management/blob/master/Dell/Manage-DellBiosPasswords-PSModule.ps1
Dell and PowerShell
Unlike HP and Lenovo, Dell does not provide command line access to their BIOS settings by default. Instead, Dell provides a PowerShell module called Dell Command | PowerShell Provider. This PowerShell module allows Dell BIOS passwords and settings to be directly viewed and modified from a PowerShell prompt or script. This PowerShell module needs to be installed before running the Dell password management script. For more information, see my recent blog post, Working with the Dell Command | PowerShell Provider.
Once the Dell PowerShell Provider module has been installed, open an administrative PowerShell prompt and import the module.
Import-Module DellBIOSProvider
Now that the module is imported, the first thing that needs to be done is to determine what passwords are currently configured. This information can be obtained using the Get-Item PowerShell cmdlet against the DellSmbios: PSDrive.
#Check the status of the admin password
Get-Item -Path DellSmbios:\Security\IsAdminPasswordSet | Select-Object -ExpandProperty CurrentValue
#Check the status of the system password
Get-Item -Path DellSmbios:\Security\IsSystemPasswordSet | Select-Object -ExpandProperty CurrentValue
Checking the status of the passwords with return either True or False.
The next step is to action on this data and set, change, or clear the password(s). This can be accomplished by using the Set-Item PowerShell cmdlet against the DellSmbios: PSDrive.
#Set a new admin password
Set-Item -Path DellSmbios:\Security\AdminPassword NewPassword
#Change an existing admin password
Set-Item -Path DellSmbios:\Security\AdminPassword NewPassword -Password OldPassword
#Clear an existing admin password
Set-Item -Path DellSmbios:\Security\AdminPassword "" -Password OldPassword
For more information on using the Dell PowerShell Provider, refer to the official documentation: Reference Guide, User Guide
Manage-DellBiosPasswords.ps1
This script takes the basic commands we just looked at and adds logic to allow for a more automated password management process. The script accepts parameters that tell it which actions to perform.
- AdminSet – Set a new admin password or change an existing admin password
- AdminClear – Clear an existing admin password
- SystemSet – Set a new system password or change an existing system password
- SystemClear – Clear an existing system password
There are also parameters that are used to specify the new and old BIOS passwords.
- AdminPassword – The current admin password or password to be set
- OldAdminPassword – The old admin password(s) to be changed. Multiple old passwords can be specified (separated by a comma).
- SystemPassword – The current system password or password to be set
- OldSystemPassword – The old system password(s) to be changed. Multiple old passwords can be specified (separated by a comma).
By default, if the script fails to perform any of these actions, it will display a message box on the screen and exit with an error code. This can be useful in a task sequence scenario where you may not want a system to continue with the task sequence if the BIOS password is not set correctly. However, if you want the script to be completely silent, there are a few parameters that can be set.
- NoUserPrompt – Suppress all user prompts
- ContinueOnError – Ignore any errors caused by changing or clearing passwords
When the script runs, it will write to a log file. By default, this log file will be named Manage-DellBiosPasswords-PSModule.Log. If the script is being run during a task sequence, the log file will be located in the _SMSTSLogPath. Otherwise, the log file will be located in ProgramData\ConfigJonScripts\Dell. The log file name and path can be changed using the LogFile parameter. Note that the log file path will always be set to _SMSTSLogPath when run during a task sequence.
Examples
The script can be run as a standalone script in Windows, or as a part of a Configuration Manager task sequence. It can also be run in the full Windows OS or in WinPE.
Here are a few examples of calling the script from a PowerShell prompt in Windows.
Set a new admin password
Manage-DellBiosPasswords-PSModule.ps1 -AdminSet -AdminPassword <String>
Set or change a admin password
Manage-DellBiosPasswords-PSModule.ps1 -AdminSet -AdminPassword <String> -OldAdminPassword <String1>,<String2>,<String3>
Set a new admin password and set a new system password
Manage-DellBiosPasswords-PSModule.ps1 -AdminSet -SystemSet -AdminPassword <String> -SystemPassword <String>
Clear an existing admin password
Manage-DellBiosPasswords-PSModule.ps1 -AdminClear -OldAdminPassword <String1>,<String2>,<String3>
Here is a basic example of calling the script during a task sequence. This is likely one of the most common ways the script would be called in a task sequence. In this example the admin password will be set if it doesn’t exist, and it will be changed if it does already exist.
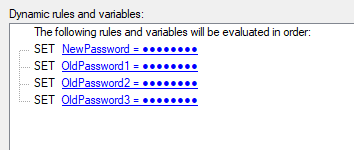

-AdminSet -AdminPassword %NewPassword% -OldAdminPassword %OldPassword1%,%OldPassword2%,%OldPassword3%
Note that before running the Dell password management script, the DellBIOSProvider PowerShell module must be installed. See my recent post Working with the Dell Command | PowerShell Provider for information on installing the module during a task sequence.
Password Lockout
If you have read my posts on managing HP and Lenovo BIOS passwords, then you will know that those scripts have logic built-in to handle system reboots. This is because on HP and Lenovo systems, after a set number of failed password attempts, the system locks and needs to be rebooted before any additional password attempts can be made.
In my testing on Dell hardware, it seems that there is not a BIOS password lockout (or it is set to a very high number) when using the Dell PowerShell Provider. This means that there isn’t a need for multiple passes of the script when there are many potential old passwords to change. The OldAdminPassword and OldSystemPassword parameters could accept many values in a single pass of the script.
Having said that, I have only tested this on a limited number of Dell hardware models, so I am not sure if it is universal. Dell could also choose to add a lockout in the future. For these reasons, I have still included the reboot logic in the script, even if it isn’t needed right now.
If multiple passes of the script are required, it would function just like the HP and Lenovo scripts.
First in the Set Password Values step, create variables for each password.
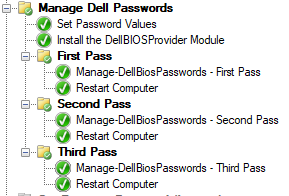
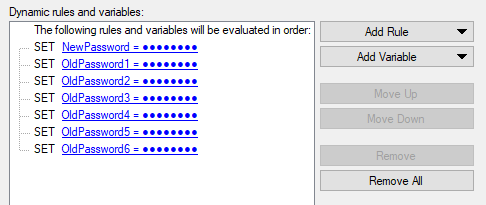
The First Pass folder has no conditions on it, as it should run for any Dell system. To set a new admin password or change an existing admin password, the AdminSet parameter is specified along with the AdminPassword and OldAdminPassword parameters.
Because the script will need to run multiple times, there is one additional parameter that needs to be specified. The SMSTSPasswordRetry parameter instructs the script to not display prompts to the screen until all attempts have completed. In this scenario, the script needs to be run 3 times, so SMSTSPasswordRetry parameter is specified on the first 2 passes of the script and not on the final pass. When the password(s) are successfully changed or cleared, the SMSTSPasswordRetry variable will be set to false. This means that if the first pass of the script is successful, the second and third passes of the script will be skipped.

-AdminSet -AdminPassword %NewPassword% -OldAdminPassword %OldPassword1%,%OldPassword2% -SMSTSPasswordRetry
When the script runs during a task sequence, it will create task sequence variables to track the success or failure of each different script action. If any one of the password actions fails, the associated task sequence variable will be set to Failed.
- DellSetAdmin
- DellClearAdmin
- DellSetSystem
- DellClearSystem
As I mentioned before, the first run of the script in the task sequence does not have any conditions, but each successive run of the script should have these conditions.
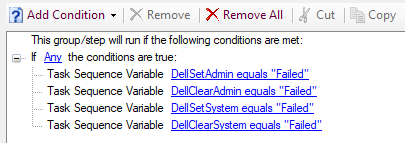
The second pass of the script. Notice the SMSTSPasswordRetry parameter is specified because there is still another potential pass of the script yet to run.

-AdminSet -AdminPassword %NewPassword% -OldAdminPassword %OldPassword3%,%OldPassword4% -SMSTSPasswordRetry
The third pass of the script. Notice the SMSTSPasswordRetry parameter is not set because this is the final pass of the script.
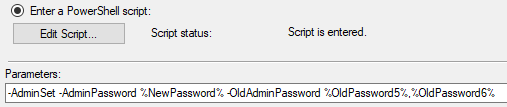
-AdminSet -AdminPassword %NewPassword% -OldAdminPassword %OldPassword5%,%OldPassword6%
User Prompts
If at the end of the script execution (whether that be one pass or multiple) any of the password management tasks are in a failed state, and the NoUserPrompt or ContinueOnError switches have not been specified, a prompt will be displayed on the screen informing the user of which tasks failed.
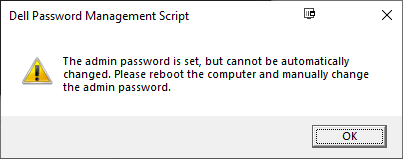
The end result of all of this is a script that can be used to change or clear Dell BIOS passwords. The script can be run in a task sequence and persist information across multiple reboots (if required). This allows for the user to be correctly prompted about any required manual actions even if there are many old passwords to test.
Notes and Additional Reading
A few notes about some restrictions Dell has placed on setting passwords.
- If a system or hard drive password is currently set, an admin password cannot be set
- If an admin password is set and a system password is not set, the admin password is required to set the system password
- If an admin password and system password are both set and the admin password is cleared, the system password will also be automatically cleared
Some Dell systems have the ability to set hard drive password(s). This script currently does not support hard drive passwords. Only admin and system passwords can be managed. I may work on adding hard drive password support in the future.
If you’re looking to configure Dell BIOS settings other than just the passwords, check out these links. Dell Command Configure, Dell PowerShell Provider User Guide, Dell PowerShell Provider Reference Guide. Also, look for a future blog post from me on configuring Dell BIOS settings with PowerShell.