The out of the box Windows 10 experience has improved over the years, but I still find most clients I work with want to make various tweaks and modifications to the default settings. In the past I would add these customizations to a Configuration Manager task sequence as individual steps. But I’m lazy, and I didn’t want to have to continually add each individual step over and over. Plus I like to keep task sequences shorter when I can. The solution was to create a PowerShell script that allows the admin to select which of the common Windows customizations to run in their environment.
There are two parts to the solution. The Parameters.ini file is where the admin makes their customization selections. The Customize_Windows.ps1 file imports data from the Parameters.ini file and then actions on that data.
Here is a snippet from the Parameters.ini file.
#Edge desktop shortcut. (1 = Remove shortcut) / (Not Configured (DEFAULT SETTING))
EdgeDesktopShortcut=1
#
#Default file explorer view. (1 = This PC) (2 = Quick Access) / (Not Configured (DEFAULT SETTING))
#FileExplorerView=1
#
#"Run as different user" in the Start menu. (1 = Show "Run As" in Start) / (Not Configured (DEFAULT SETTING))
RunAsUserStart=1
#
Any line in the parameters file that starts with a # will be ignored by the main script. Any lines without a # at the beginning will be imported by the main script. A variable will be created with a name matching the string on the left side of the = sign. The value for that variable will be set to the string on the right side of the = sign.
In this example, a variable named EdgeDesktopShortcut will be created and set to a value of 1. Another variable named RunAsUserStart will be created and set to a value of 1. The FileExplorerView line will be ignored, as it begins with a #. The result of running the script will be the Edge desktop shortcut being deleted and the “Run as different user” option being accessible in the Start menu.
Here is a snippet from the PowerShell script showing the customizations being run if the variable is created.
if ($NULL -ne $EdgeDesktopShortcut)
{
New-RegistryValue -Customization "Edge Desktop Shortcut" -RegKey "HKLM:\SOFTWARE\Microsoft\Windows\CurrentVersion\Explorer" -Name "DisableEdgeDesktopShortcutCreation" -PropertyType DWord -Value $EdgeDesktopShortcut
}
if ($NULL -ne $FileExplorerView)
{
New-RegistryValue -Customization "File Explorer View" -RegKey "HKLM:\SOFTWARE\Microsoft\Windows\CurrentVersion\Explorer\Advanced" -Name "LaunchTo" -PropertyType DWord -Value $FileExplorerView
}
if ($NULL -ne $RunAsUserStart)
{
New-RegistryValue -Customization "Run As User Start Menu" -RegKey "HKLM:\SOFTWARE\Policies\Microsoft\Windows\Explorer" -Name "ShowRunasDifferentuserinStart" -PropertyType DWord -Value $RunAsUserStart
}
The majority of the customizations are registry modifications. However, there are a couple of options that warrant a quick explanation. The StartLayout and DefaultApps options in the Parameters.ini file require a .xml file name as input. The script will attempt to locate the specified files in the root directory of the PowerShell script. If using these options ensure all files are saved in the same directory. For more information on how to generate these files, see these links: Start Layout, Default Apps.
The PowerShell script has 1 parameter, -File, which is used to point to the Parameters.ini file. The script can be called during a Configuration Manager task sequence using a Run PowerShell Script step.
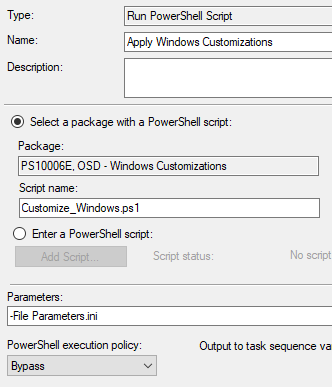
The full PowerShell script and a Parameters.ini file can be downloaded from my GitHub. https://github.com/ConfigJon/Windows-Customizations/tree/master/Customization-Script